On my programming blog, I often try to compare performance characteristics of different algorithms or concepts. I usually log performance output (like elapsed time) to the console or a txt file and then copy this to a spreadsheet and analyse. But recently, I've found another way of doing this: I've used Spire.XSL library to generate the final spreadsheet file - with all the tables and charts! Read further to learn how one can leverage this library for various automation tasks.
Download C# project - 7.1 KB, zip
The Case
Our objective is to create a benchmark application that will test three different sorting algorithms. We would like to get elapsed time for a different element count. Below, there is a simple code that can be used:
abstract class PerfTestBase
{
public double ElapsedTimeSec {get; protected set;}
public string Name {get; protected set;}
public abstract void run(int n);
}
class BubbleSortPerfTest : PerfTestBase
{
public BubbleSortPerfTest()
{
Name = "Bubble Sort";
}
public override void run(int n)
{
// real implementation here
ElapsedTimeSec = X;
}
}
class MergeSortPerfTest : PerfTestBase
{
public MergeSortPerfTest()
{
Name = "Merge Sort";
}
public override void run(int n)
{
// real implementation here
ElapsedTimeSec = X;
}
}
class QuickSortPerfTest : PerfTestBase
{
public QuickSortPerfTest()
{
Name = "Quick Sort";
}
public override void run(int n)
{
// real implementation here
ElapsedTimeSec = X;
}
}
Algorithms are ready and now we need to run them with different startup parameters.
List<PerfTestBase> perfTests = new List<PerfTestBase>
{
new BubbleSortPerfTest(),
new MergeSortPerfTest(),
new QuickSortPerfTest()
};
// N from 10 up to 200, step is 10
var res = runAllTests(perfTests, 10, 200, 10);
printResults(res);
The function runAllTests
simply iterates through set of N
values and calls .run(N)
methods.
The most interesting part for us is the printResults
method. What code can be used to automate reporting and generate valuable results?
Simplest Solution
Initially we can, of course, print all the results to the console. We can even use CSV format and then easily copy it to spreadsheet.
N;Bubble Sort;Merge Sort;Quick Sort;
10;20,00;140,46;96,71;
20;80,00;365,48;251,64;
After a while, when you continue to change your algorithm code, the task of copying results becomes tedious. For sure, there must be a better and faster way. What if we could generate not CSV file, but full Excel file? And now is a best place to introduce our Spire.XLS library.
Introducing Spire.XLS
Spire.XSL is a library that makes Office automation easier.
But briefly: Add reference to Spire.XLS in your project and then you can create, open, update, run calculation without requiring Microsoft Excel or Microsoft Office to be installed on the system!
The library is fully compatible with Excel 97/2003, 2007 and 2010.
Additionally Spire.XLS can also protect, encrypt files and, what is more important, convert to other formats. You can for instance export your files to PDF, images or HTML.
This solution gives us possibility to implement valuable and automated applications quite easily.
Using Spire.XSL in the Code
In our example, we will use probably only 1% of the full power of the library! Still it will save us a lot of time with report generation.
Basic Usage
Add references:
using Spire.Xls;
using Spire.Xls.Charts;
Four lines to create 'Hello World' workbook:
Workbook wb = new Workbook();
Worksheet sheet = wb.Worksheets[0];
sheet.Range["A1"].Text = "Hello,World!";
wb.SaveToFile("Sample.xls",
ExcelVersion.Version2007);
The above code gives a basic idea how the library looks like. Basically you can manipulate workbooks, sheets and individual cells in a very light way.
Improved Solution
Let's go back to our original problem. Our new solution will keep the console output part, but we will save the results also to Excel file. In addition to that, we can create a chart. That way, a lot of time will be saved - no need for copy and regenerate charts again and again...
Here is a fragment of code related to saving the data:
Worksheet sheet = workbook.Worksheets[0];
sheet.Name = "Perf Test";
sheet.Range["A1"].Text = "Elapsed Time for sorting...";
sheet.Range["A1"].Style.Font.IsBold = true;
// columns title:
sheet.Range["C3"].Text = "N";
sheet.Range["C3"].Style.Font.IsBold = true;
sheet.Range["C3"].Style.HorizontalAlignment = HorizontalAlignType.Center;
char col = 'D';
foreach (var n in res.Map.Keys)
{
sheet.Range[col+"3"].Text = n;
sheet.Range[col+"3"].Style.Font.IsBold = true;
sheet.Range[col+"3"].Style.HorizontalAlignment = HorizontalAlignType.Center;
col++;
}
// insert values into rows...
And here is some of the chart generation code:
Chart chart = sheet.Charts.Add();
//Set region of chart data
chart.DataRange = workbook.Worksheets[0].Range[range];
chart.SeriesDataFromRange = false;
//Set position of chart
chart.LeftColumn = 2;
chart.TopRow = 2;
chart.RightColumn = 12;
chart.BottomRow = 30;
//Chart title
chart.ChartTitle = "Sorting Time...";
chart.ChartTitleArea.IsBold = true;
chart.ChartTitleArea.Size = 12;
// ...
chart.Legend.Position = LegendPositionType.Bottom;
chart.ChartType = ExcelChartType.ScatterSmoothedLineMarkers;
Simple as it is!
I especially like the way we can get to a cell or a whole range. Note how easy it is to change style of a cell.
A final Excel file - generate automatically of course:
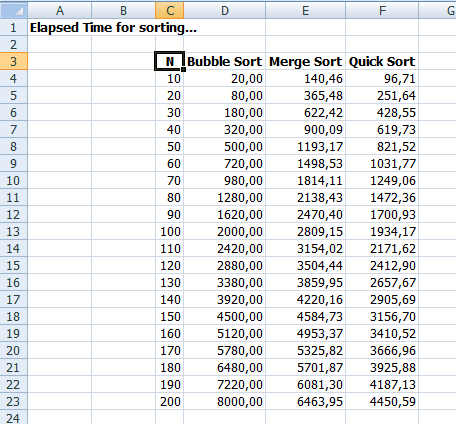
and the chart:
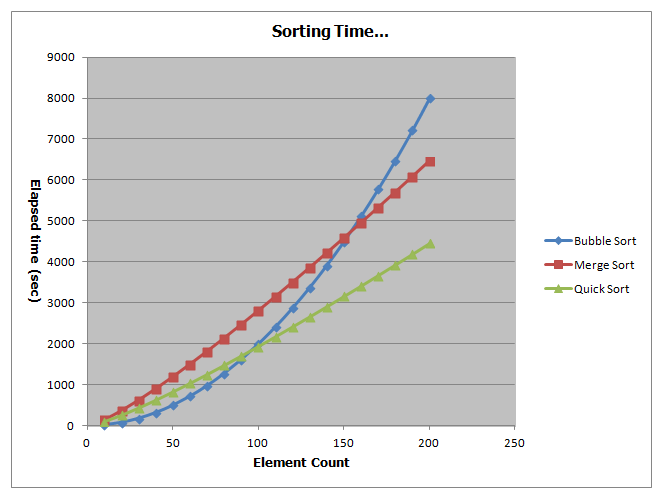
Alternatives
If you want to go opensource:
- ClosedXML - ClosedXML allows you to create Excel 2007/2010 files without the Excel application
- EPPlus - library that reads and writes Excel 2007/2010 files using the Open Office Xml format (xlsx)
- NPOI - an open source project which can help you read/write xls, doc, ppt files.
Summary
In this article, I've shown how we can easily automate the task of reporting performance results from an application. By using Spire.XLS, programmers are able to create and manipulate Excel files without having Office installation on the system. The library is very powerful and, what is more important, trivial to utilize. Our task - creating reports - could be automated in a few lines of code.
Remarks
- The library is designed for .NET, but even in native code we could use the same solution. I need to test this, but we could create a 'bridge' and call .NET library from C++ application. C++ app will do the work, but all the results would go to .NET module that will call Spire.XLS.
Article was sponsored by e-iceblue company.