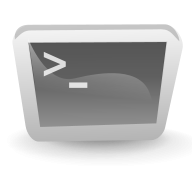
For most of the time I use some IDE (like Visual Studio) to build the software. It is very convenient and improves programming, but what would happen when I had only the command line and a simple text editor? Let's try!
This is a great method for doing some review of one's programming knowledge. IDE offers sometimes too much automatization and it is easy to forget (or do not bother) what is going on under the hood.Setup:
- Windows
- MinGW (g++, gdb)
- MSys (make)
- Notepad++
g++
G++ is part of the GCC. When using on Windows you have to download MinGW or Cygwin. MinGW seems to be a bit easier to use (although not so advanced as Cygwin). It is worth to mention that Cygwin generates code that is compatible with POSIX standard, MinGW not.The simplest way to compile and build the app from source and from command line:
// this will build test.exe from test.cpp file
g++ -Wall -g test.cpp -o test
// -Wall - show warnings
// -g - generate debug info for GDB
// -DDEBUG - define DEBUG preprocessor flag
links:
- gcc-intro.html - basic tutorial and introduction
- gcc/Option-Index.html - list of all options for gcc
gdb
This is quite powerful gcc debugger. It works from command line but various IDE adds even 'visual' debugging mode built on top of gdb. Remember to add '-g' when compiling - that way program will have debug information embedded in the binary.
gdb appName - start the session (this will move us to gdb command line mode)
run - run the app
b 12 - set breakpoint at line 12
bt funcName - set breakpoint at given function
p dd - display value of the 'dd' variable
s - step into function
n - next go over the next step
links:
make:
It would be very hard to enter gcc command line options every time you need to build an app. For simple projects one can try that simple solution but after a while it becomes boring and error prone. MAKE is a powerful tool that is used mostly to build code. You need to write a script and then 'execute' this in MAKE. Orginally MAKE comes from linux/unix tools but now it is widely available in most of OS. For this post I used make that comes with MSys (additional tools for MinGW)
target: dependenciesAnd here is my awesome makefile that I use to build my examples:
[tab] system command
CC=g++
INCLUDES=../utils/
CFLAGS=-Wall -W -I $(INCLUDES)
CFLAGS_BOOST=$(CFLAGS) -I"$(BOOST_DIR)"
ifeq "$(debug)" "1"
CFLAGS += -DDEBUG -g
endif
ifeq "$(cpp11)" "1"
CFLAGS += -std=c++0x
endif
all: searching pointers lambda
.PHONY : clean
clean:
rm -f *.exe
searching: CFLAGS += -std=c++0x
searching: searching.cpp
$(CC) $(CFLAGS) $< -o $@
pointers: CFLAGS += -std=c++0x
pointers: pointers.cpp
$(CC) $(CFLAGS) $< -o $@
lambda: CFLAGS += -std=c++0x
lambda: lambda.cpp
$(CC) $(CFLAGS) $< -o $@
This makefile basically creates execs for three projects: searching, pointers, lambda. In the beginning there are some variables and globals that are used for the build process. There are CFLAGS, include dirs and options for cpp11 and debug mode.
At the end there are targets to build. If I decide to create another test application that will be placed in the same directory I need to put only these lines into the makefile
At the end there are targets to build. If I decide to create another test application that will be placed in the same directory I need to put only these lines into the makefile
links:
myNewApp : myNewApp.cpp
$(CC) $(CFLAGS) $< -o $@
// plus add myNewApp to 'all' target
- http://mrbook.org/tutorials/make/ (very simple but useful and gives quick start)
- http://www.opussoftware.com/tutorial/TutMakefile.ht - a bit longer then the previous link
- http://makepp.sourceforge.net/1.19/makepp_tutorial.htm - quite long tutorial
- http://www.gnu.org/software/make/manual/make.html - full gnu make reference
Improvements
- option: use Linux (even from a virtual machine)
- another hardcore option: use VI(M)
image from: openclipart.org